feedbackとは
feedback
はFlutterアプリケーションでインタラクティブなフィードバックを取得するためのパッケージです。
ユーザーが現在のページのスクリーンショットを注釈付きで提供し、テキストも追加できるようにします。
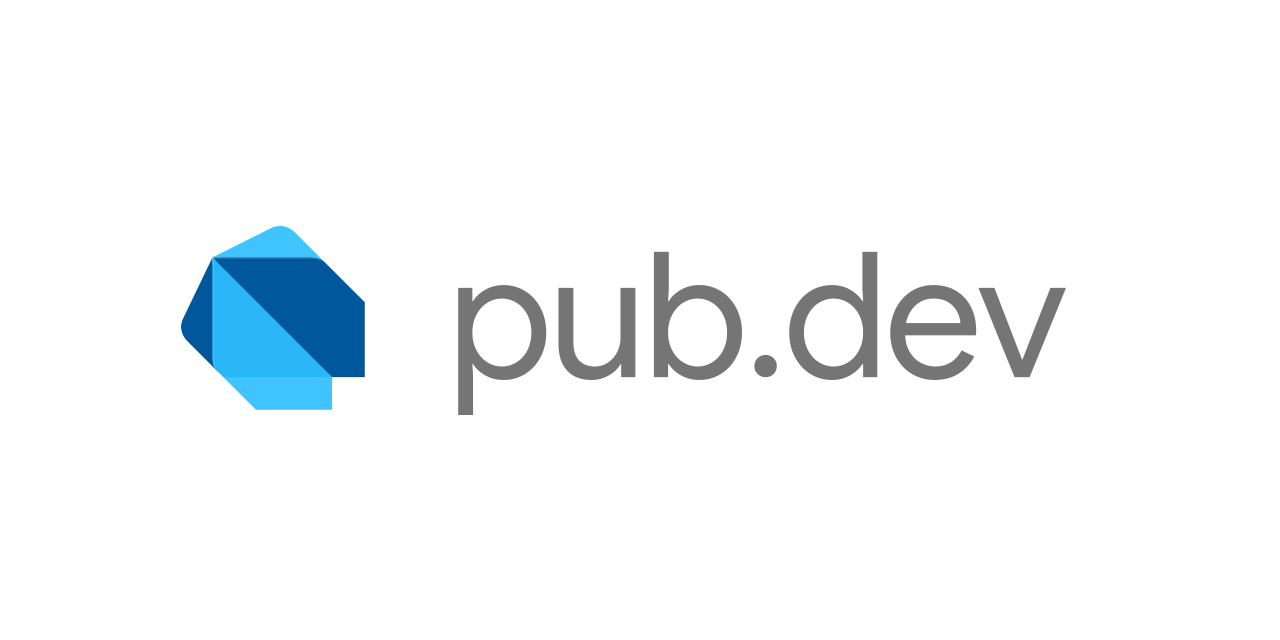
feedback | Flutter package
A Flutter package for getting better feedback. It allows the user to give interactive feedback directly in the app.
使い方
セットアップ
dependencies:
flutter:
sdk: flutter
feedback: ^3.1.0
基本的な使い方
- アプリを
BetterFeedback
ウィジェットでラップします。 - フィードバックビューを表示するために
BetterFeedback.of(context).show(...)
を呼び出します。
import 'package:feedback/feedback.dart';
import 'package:flutter/material.dart';
void main() {
runApp(
BetterFeedback(
child: const MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Feedback Example'),
),
body: Center(
child: ElevatedButton(
onPressed: () {
BetterFeedback.of(context).show((UserFeedback feedback) {
// フィードバックを処理する
print('Feedback: ${feedback.text}, Screenshot: ${feedback.screenshot}');
});
},
child: Text('Give Feedback'),
),
),
),
);
}
}
フィードバックパネルの表示と非表示
フィードバックパネルを表示する方法:
BetterFeedback.of(context).show((UserFeedback feedback) {
// フィードバックを処理する
print('Feedback: ${feedback.text}, Screenshot: ${feedback.screenshot}');
});
フィードバックパネルを非表示にする方法:
BetterFeedback.of(context).hide();
フィードバックの利用方法
フィードバックを活用するためのさまざまなソリューションがあります。以下はその一例です。
GitLabプラグイン
feedback_gitlab
プラグインは、ユーザーが送信したフィードバックごとにGitLabにイシューを作成します。
import 'package:feedback_gitlab/feedback_gitlab.dart';
BetterFeedback.of(context).showAndUploadToGitLab(
projectId: 'your_project_id', // 必須、GitLabプロジェクトID
apiToken: 'your_api_token', // 必須、GitLab APIトークン
gitlabUrl: 'gitlab.org', // オプション、デフォルトは'gitlab.com'
);
Sentryプラグイン
feedback_sentry
プラグインは、フィードバックをSentryにSentry User Feedbackとして送信します。
import 'package:feedback_sentry/feedback_sentry.dart';
BetterFeedback.of(context).showAndUploadToSentry(
name: 'Foo Bar', // オプション
email: 'foo_bar@example.com', // オプション
);
その他のユースケース
フィードバックをサーバーにアップロードする場合、MultipartRequest
などを使用します。
プラットフォーム共有ダイアログを使用して共有する場合、share_plus
パッケージを使用します。
Firebase、Jira、Trello、Eメールなどの他のサービスにアップロードすることも可能です。
カスタマイズと設定
フィードバックテーマやローカリゼーションのカスタマイズも可能です。
テーマの設定
import 'package:feedback/feedback.dart';
import 'package:flutter/material.dart';
void main() {
runApp(
BetterFeedback(
child: const MyApp(),
theme: FeedbackThemeData(
background: Colors.grey,
feedbackSheetColor: Colors.grey[50]!,
drawColors: [
Colors.red,
Colors.green,
Colors.blue,
Colors.yellow,
],
),
),
);
}
ローカリゼーションのカスタマイズ
class CustomFeedbackLocalizations implements FeedbackLocalizations {
// カスタムローカリゼーションの実装
}
class CustomFeedbackLocalizationsDelegate extends GlobalFeedbackLocalizationsDelegate {
static final supportedLocales = <Locale, FeedbackLocalizations>{
const Locale('en'): const CustomFeedbackLocalizations(),
};
}
void main() {
runApp(
BetterFeedback(
child: const MyApp(),
localizationsDelegates: [
GlobalMaterialLocalizations.delegate,
GlobalCupertinoLocalizations.delegate,
GlobalWidgetsLocalizations.delegate,
CustomFeedbackLocalizationsDelegate(),
],
),
);
}