firebase_authとは
firebase_auth
は、Firebase認証APIを使用するためのFlutterプラグインです。このプラグインを使用することで、パスワード、電話番号、Google、Facebook、Twitterなどの認証プロバイダを利用した認証が可能になります。firebase_auth
を使用すると、ユーザーの登録、ログイン、認証ステータスの管理など、さまざまな認証機能を簡単に実装できます。
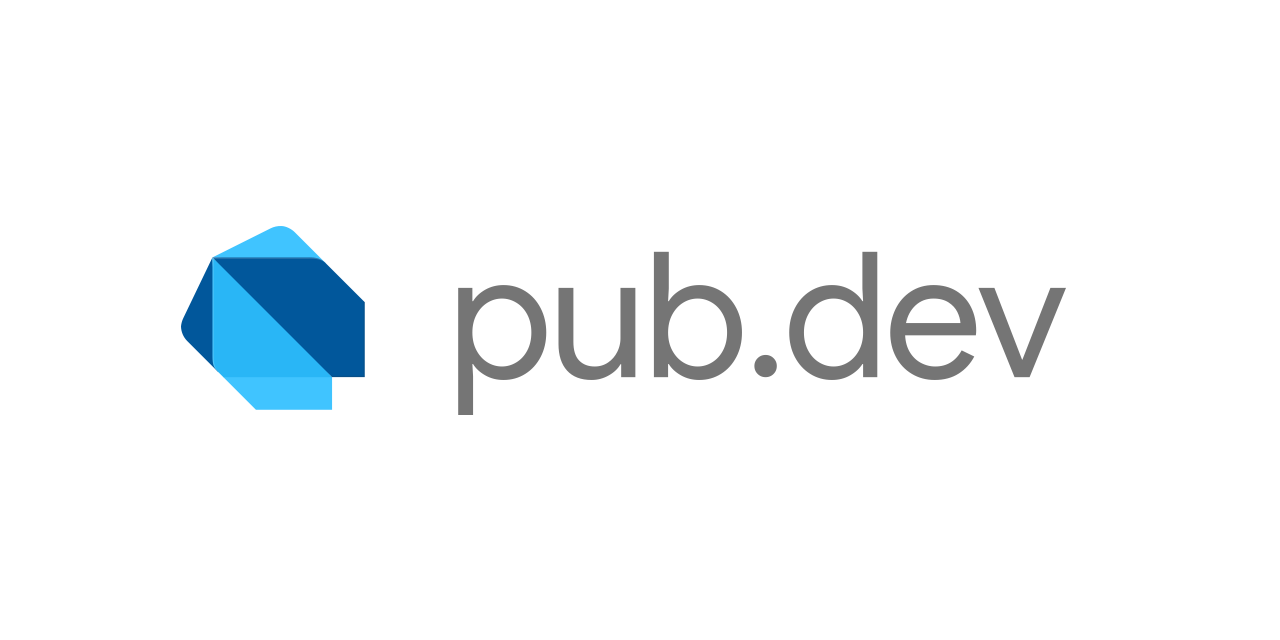
firebase_auth | Flutter package
Flutter plugin for Firebase Auth, enabling authentication using passwords, phone numbers and identity providers like Goo...
使い方
インストール方法
pubspec.yaml
ファイルに依存関係を追加します。
dependencies:
firebase_auth: ^4.20.0
Firebaseプロジェクトの設定
Firebaseプロジェクトを作成し、Flutterアプリと連携する必要があります。以下は、Firebaseプロジェクトの設定手順の概要です。
- Firebaseコンソールにアクセスし、新しいプロジェクトを作成します。
- プロジェクトにアプリ(iOS、Android、Webなど)を追加し、Firebase SDKを設定します。
google-services.json
(Android)またはGoogleService-Info.plist
(iOS)ファイルをFlutterプロジェクトに追加します。
詳細な手順については、公式ドキュメントを参照してください。
基本的な使用方法
ユーザーの登録
Future<User?> registerWithEmailAndPassword(String email, String password) async {
try {
UserCredential userCredential = await FirebaseAuth.instance.createUserWithEmailAndPassword(
email: email,
password: password,
);
return userCredential.user;
} on FirebaseAuthException catch (e) {
print('Failed to register: $e');
return null;
}
}
ユーザーのログイン
Future<User?> signInWithEmailAndPassword(String email, String password) async {
try {
UserCredential userCredential = await FirebaseAuth.instance.signInWithEmailAndPassword(
email: email,
password: password,
);
return userCredential.user;
} on FirebaseAuthException catch (e) {
print('Failed to sign in: $e');
return null;
}
}
ユーザーのサインアウト
Future<void> signOut() async {
await FirebaseAuth.instance.signOut();
}
認証状態の監視
FirebaseAuth.instance.authStateChanges().listen((User? user) {
if (user == null) {
print('User is currently signed out!');
} else {
print('User is signed in!');
}
});
パラメータ詳細
createUserWithEmailAndPassword
email
:新しいユーザーのメールアドレス。password
:新しいユーザーのパスワード。
signInWithEmailAndPassword
email
:既存のユーザーのメールアドレス。password
:既存のユーザーのパスワード。
signOut
- 戻り値なし。現在のユーザーをサインアウトします。
エラー処理
Firebase認証操作は多くの場合、エラーが発生する可能性があります。例えば、既に登録されているメールアドレスでの登録試行や、無効なメールアドレスでのログイン試行などです。これらのエラーは、FirebaseAuthException
でキャッチされ、適切に処理する必要があります。
try {
// 認証操作
} on FirebaseAuthException catch (e) {
switch (e.code) {
case 'weak-password':
print('The password provided is too weak.');
break;
case 'email-already-in-use':
print('The account already exists for that email.');
break;
default:
print('Authentication error: ${e.message}');
}
}