animated_text_kitとは
animated_text_kit
は、Flutterアプリケーションにおけるテキストアニメーションを簡単に実装できるパッケージです。
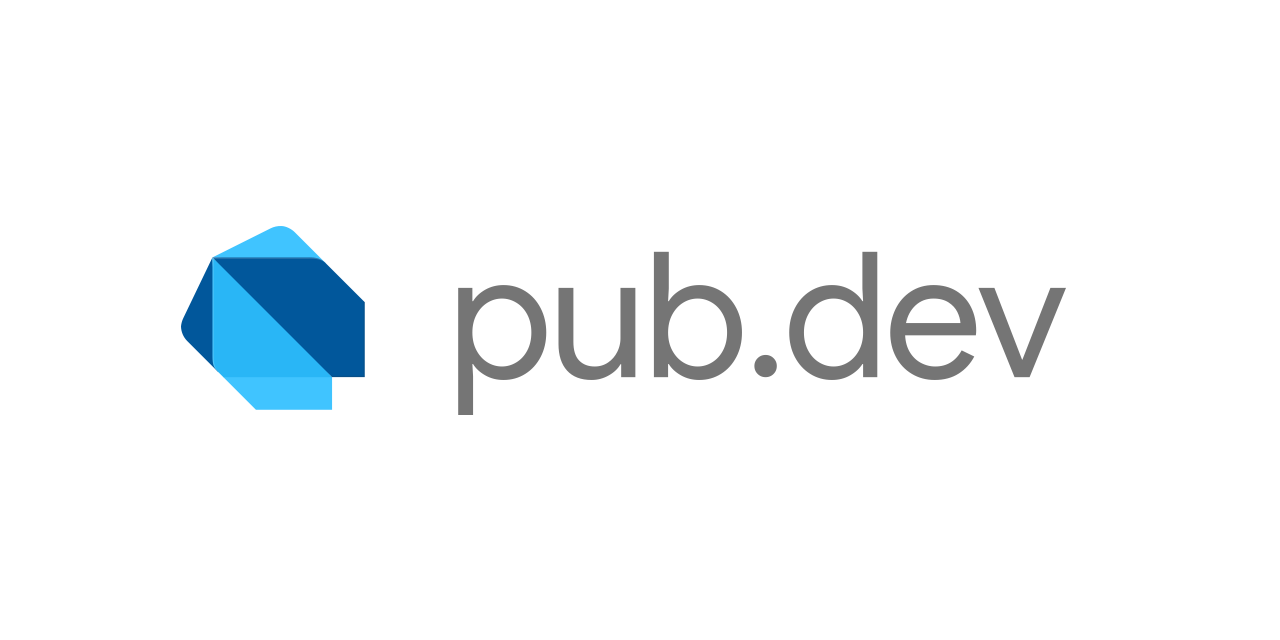
animated_text_kit | Flutter package
A flutter package project which contains a collection of cool and beautiful text animations.
使い方
インストール方法
pubspec.yaml
ファイルに依存関係を追加します。
dependencies:
animated_text_kit: ^4.2.2
基本的な使い方
以下は、TypewriterAnimatedText
を使用した簡単な例です。
AnimatedTextKit(
animatedTexts: [
TypewriterAnimatedText(
'Hello world!',
textStyle: const TextStyle(
fontSize: 32.0,
fontWeight: FontWeight.bold,
),
speed: const Duration(milliseconds: 2000),
),
],
totalRepeatCount: 4,
pause: const Duration(milliseconds: 1000),
displayFullTextOnTap: true,
stopPauseOnTap: true,
)
パラメータの詳細
animatedTexts
: 使用するアニメーションテキストのリストを指定します。totalRepeatCount
: アニメーションを繰り返す回数を指定します(repeatForever
がfalse
の場合)。pause
: 各アニメーションテキスト間の一時停止時間を指定します。displayFullTextOnTap
: タップでアニメーションを完了させるかどうかを指定します。stopPauseOnTap
: タップで一時停止を解除するかどうかを指定します。isRepeatingAnimation
: アニメーションを繰り返すかどうかを指定します。repeatForever
: アニメーションを永遠に繰り返すかどうかを指定します。
コールバック
onTap
: ユーザーがアニメーションテキストをタップしたときに呼ばれます。onNext(int index, bool isLast)
: 前のテキストの一時停止後、次のテキストアニメーションの前に呼ばれます。onNextBeforePause(int index, bool isLast)
: 前のテキストの一時停止前、次のテキストアニメーションの前に呼ばれます。onFinished
: アニメーションが終了したときに呼ばれます(isRepeatingAnimation
がfalse
の場合)。
アニメーションの例
Rotate
Row(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
const SizedBox(width: 20.0, height: 100.0),
const Text(
'Be',
style: TextStyle(fontSize: 43.0),
),
const SizedBox(width: 20.0, height: 100.0),
DefaultTextStyle(
style: const TextStyle(
fontSize: 40.0,
fontFamily: 'Horizon',
),
child: AnimatedTextKit(
animatedTexts: [
RotateAnimatedText('AWESOME'),
RotateAnimatedText('OPTIMISTIC'),
RotateAnimatedText('DIFFERENT'),
],
onTap: () {
print("Tap Event");
},
),
),
],
);
Fade
return SizedBox(
width: 250.0,
child: DefaultTextStyle(
style: const TextStyle(
fontSize: 32.0,
fontWeight: FontWeight.bold,
),
child: AnimatedTextKit(
animatedTexts: [
FadeAnimatedText('do IT!'),
FadeAnimatedText('do it RIGHT!!'),
FadeAnimatedText('do it RIGHT NOW!!!'),
],
onTap: () {
print("Tap Event");
},
),
),
);
Typer
return SizedBox(
width: 250.0,
child: DefaultTextStyle(
style: const TextStyle(
fontSize: 30.0,
fontFamily: 'Bobbers',
),
child: AnimatedTextKit(
animatedTexts: [
TyperAnimatedText('It is not enough to do your best,'),
TyperAnimatedText('you must know what to do,'),
TyperAnimatedText('and then do your best'),
TyperAnimatedText('- W.Edwards Deming'),
],
onTap: () {
print("Tap Event");
},
),
),
);
Typewriter
return SizedBox(
width: 250.0,
child: DefaultTextStyle(
style: const TextStyle(
fontSize: 30.0,
fontFamily: 'Agne',
),
child: AnimatedTextKit(
animatedTexts: [
TypewriterAnimatedText('Discipline is the best tool'),
TypewriterAnimatedText('Design first, then code'),
TypewriterAnimatedText('Do not patch bugs out, rewrite them'),
TypewriterAnimatedText('Do not test bugs out, design them out'),
],
onTap: () {
print("Tap Event");
},
),
),
);
Scale
return SizedBox(
width: 250.0,
child: DefaultTextStyle(
style: const TextStyle(
fontSize: 70.0,
fontFamily: 'Canterbury',
),
child: AnimatedTextKit(
animatedTexts: [
ScaleAnimatedText('Think'),
ScaleAnimatedText('Build'),
ScaleAnimatedText('Ship'),
],
onTap: () {
print("Tap Event");
},
),
),
);
Colorize
const colorizeColors = [
Colors.purple,
Colors.blue,
Colors.yellow,
Colors.red,
];
const colorizeTextStyle = TextStyle(
fontSize: 50.0,
fontFamily: 'Horizon',
);
return SizedBox(
width: 250.0,
child: AnimatedTextKit(
animatedTexts: [
ColorizeAnimatedText(
'Larry Page',
textStyle: colorizeTextStyle,
colors: colorizeColors,
),
ColorizeAnimatedText(
'Bill Gates',
textStyle: colorizeTextStyle,
colors: colorizeColors,
),
ColorizeAnimatedText(
'Steve Jobs',
textStyle: colorizeTextStyle,
colors: colorizeColors,
),
],
isRepeatingAnimation: true,
onTap: () {
print("Tap Event");
},
),
);
TextLiquidFill
return SizedBox(
width: 250.0,
child: TextLiquidFill(
text: 'LIQUIDY',
waveColor: Colors.blueAccent,
boxBackgroundColor: Colors.redAccent,
textStyle: TextStyle(
fontSize: 80.0,
fontWeight: FontWeight.bold,
),
boxHeight: 300.0,
),
);
Wavy
return DefaultTextStyle(
style: const TextStyle(
fontSize: 20.0,
),
child: AnimatedTextKit(
animatedTexts: [
WavyAnimatedText('Hello World'),
WavyAnimatedText('Look at the waves'),
],
isRepeatingAnimation: true,
onTap: () {
print("Tap Event");
},
),
);
Flicker
return SizedBox(
width: 250.0,
child: DefaultTextStyle(
style: const TextStyle(
fontSize: 35,
color: Colors.white,
shadows: [
Shadow(
blurRadius: 7.0,
color: Colors.white,
offset: Offset(0, 0),
),
],
),
child: AnimatedTextKit(
repeatForever: true,
animatedTexts: [
FlickerAnimatedText('Flicker Frenzy'),
FlickerAnimatedText('Night Vibes On'),
FlickerAnimatedText("C'est La Vie !"),
],
onTap: () {
print("Tap Event");
},
),
),
);
カスタムアニメーションの作成
AnimatedText
を拡張する新しいクラスを作成することで、独自のアニメーションを簡単に作成できます。必要なメソッドを実装し、AnimatedTextKit
で表示します。
AnimatedTextKit(
animatedTexts: [
CustomAnimatedText(
'Insert Text Here',
textStyle: const TextStyle(
fontSize: 32.0,
fontWeight: FontWeight.bold,
),
),
],
);