url_launcherとは
WebブラウザでURLを開いたり、メールアプリを起動したり、電話アプリを起動して電話をかけたりすることができます。
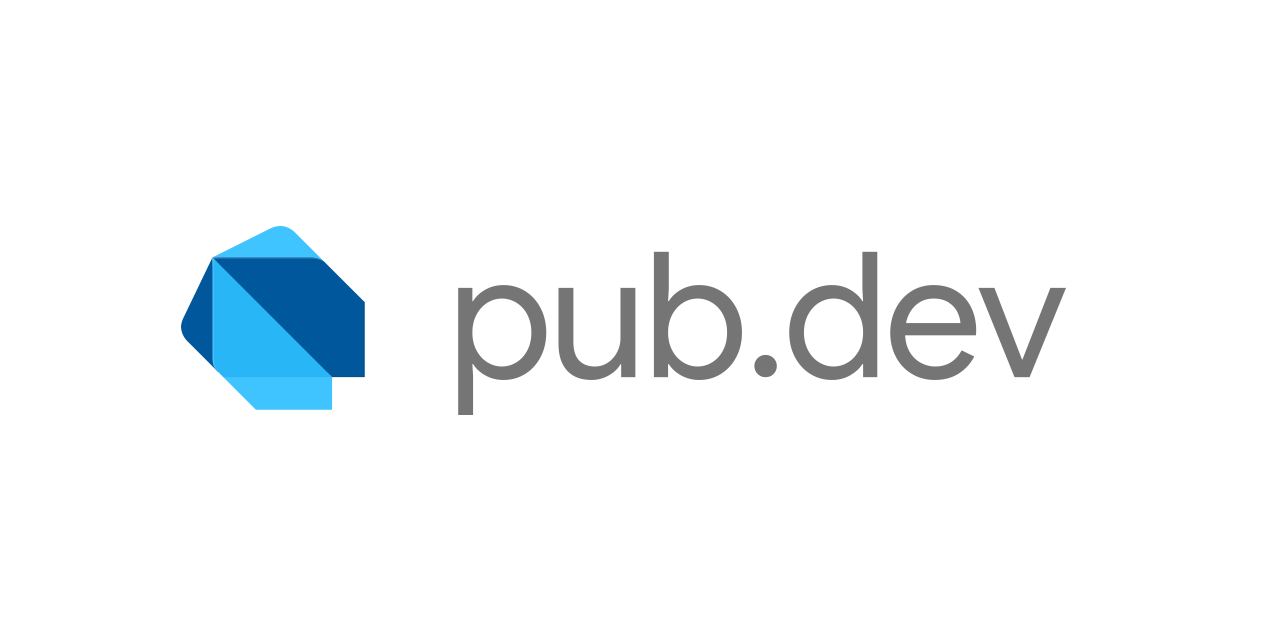
url_launcher | Flutter package
Flutter plugin for launching a URL. Supports web, phone, SMS, and email schemes.
使い方
このプラグインを使用するには、まず pubspec.yaml
ファイルに url_launcher
を依存関係として追加する必要があります。
dependencies:
flutter:
sdk: flutter
url_launcher: ^6.2.6
インポートと基本的な使用例
import 'package:flutter/material.dart';
import 'package:url_launcher/url_launcher.dart';
final Uri _url = Uri.parse('https://flutter.dev');
void main() => runApp(
const MaterialApp(
home: Material(
child: Center(
child: ElevatedButton(
onPressed: _launchUrl,
child: Text('Show Flutter homepage'),
),
),
),
),
);
Future<void> _launchUrl() async {
if (!await launchUrl(_url)) { // url_launcherを利用している箇所
throw Exception('Could not launch $_url');
}
}
詳細な設定
iOS
iOSで特定のURLスキームを使用する場合は、Info.plist
ファイルにそのスキームを追加する必要があります。
<key>LSApplicationQueriesSchemes</key>
<array>
<string>sms</string>
<string>tel</string>
</array>
Android
Androidでは、特定のURLスキームを使用する場合は、AndroidManifest.xml
ファイルにそのスキームを追加する必要があります。
<queries>
<intent>
<action android:name="android.intent.action.VIEW" />
<data android:scheme="sms" />
</intent>
<intent>
<action android:name="android.intent.action.VIEW" />
<data android:scheme="tel" />
</intent>
<intent>
<action android:name="android.support.customtabs.action.CustomTabsService" />
</intent>
</queries>
サポートされているURLスキーム
一般的に使用されるURLスキームの例は以下の通りです:
https:<URL>
:デフォルトブラウザでURLを開くmailto:<email address>?subject=<subject>&body=<body>
:デフォルトのメールアプリでメールを作成するtel:<phone number>
:デフォルトの電話アプリで電話をかけるsms:<phone number>
:デフォルトのメッセージングアプリでSMSを送信する
URLスキームのサポート確認
実行時にスキームがサポートされているか確認する場合は、canLaunchUrl
関数を使用します。ただし、canLaunchUrl
は一部の環境で false
を返す場合があるため、直接 launchUrl
を使用して失敗をハンドリングする方法が推奨されます。
Future<void> _launchUrl() async {
if (!await canLaunchUrl(_url)) {
throw Exception('Could not launch $_url');
}
await launchUrl(_url);
}
URLのエンコード
URLにスペースや特殊文字を含む場合は、適切にエンコードする必要があります。Uri
クラスは通常この処理を自動的に行いますが、特定のスキーム(例えば mailto
や sms
)では手動でエンコードする必要があります。
String? encodeQueryParameters(Map<String, String> params) {
return params.entries
.map((MapEntry<String, String> e) =>
'${Uri.encodeComponent(e.key)}=${Uri.encodeComponent(e.value)}')
.join('&');
}
final Uri emailLaunchUri = Uri(
scheme: 'mailto',
path: 'smith@example.com',
query: encodeQueryParameters(<String, String>{
'subject': 'Example Subject & Symbols are allowed!',
}),
);
launchUrl(emailLaunchUri);