share_plusとは
コンテンツを他のアプリやサービスと共有するためのプラグインです。
テキスト、ファイル、URLなどを簡単に共有することができます。
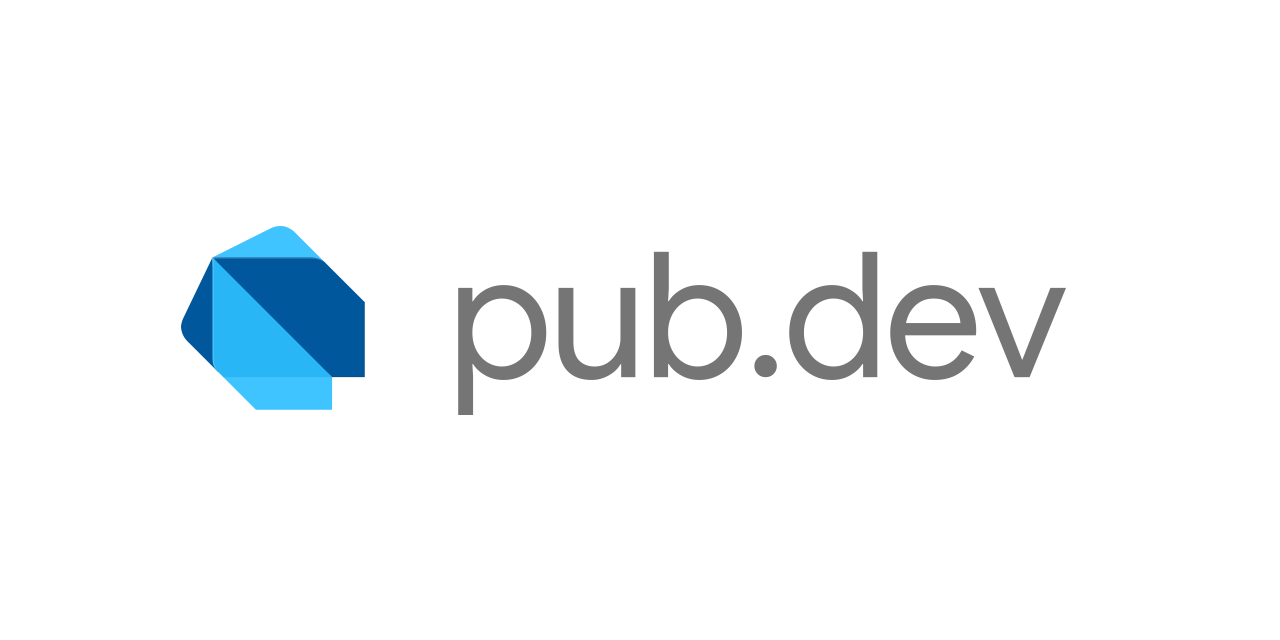
share_plus | Flutter package
Flutter plugin for sharing content via the platform share UI, using the ACTION_SEND intent on Android and UIActivityView...
使い方
dependencies:
flutter:
sdk: flutter
share_plus: ^9.0.0
インポートと基本的な使用例
import 'package:share_plus/share_plus.dart';
テキストを共有
テキストを共有するには、Share.share
メソッドを使用します。
Share.share('check out my website https://example.com');
オプションで、共有時に使用される subject
を指定することもできます。
Share.share('check out my website https://example.com', subject: 'Look what I made!');
共有結果の確認
共有シートでユーザーアクションの結果を確認することも可能です。
final result = await Share.share('check out my website https://example.com');
if (result.status == ShareResultStatus.success) {
print('Thank you for sharing my website!');
}
ファイルを共有
1つまたは複数のファイルを共有するには、Share.shareXFiles
メソッドを使用します。
final result = await Share.shareXFiles([XFile('${directory.path}/image.jpg')], text: 'Great picture');
if (result.status == ShareResultStatus.success) {
print('Thank you for sharing the picture!');
}
final result = await Share.shareXFiles([XFile('${directory.path}/image1.jpg'), XFile('${directory.path}/image2.jpg')]);
if (result.status == ShareResultStatus.dismissed) {
print('Did you not like the pictures?');
}
Webでの使用
Webでは、Share.shareXFiles
を使用してファイルを共有できます。Web Share APIが利用できない場合は、ファイルのダウンロードにフォールバックします。
Share.shareXFiles([XFile('assets/hello.txt')], text: 'Great picture');
URIの共有
iOSでは、URIからメタデータを取得して共有することがサポートされています。このためには、Share.shareUri
メソッドを使用します。
Share.shareUri(uri: uri);
共有結果の取得
すべての共有メソッドは、ShareResult
オブジェクトを返します。このオブジェクトには、次の情報が含まれます。
status
: 共有結果を示すShareResultStatus
raw
: 共有結果を説明する文字列(例:開いているアプリのID)
final result = await Share.share('check out my website https://example.com');
if (result.status == ShareResultStatus.success) {
print('Thank you for sharing!');
} else if (result.status == ShareResultStatus.dismissed) {
print('Sharing was dismissed.');
}
iPadでの共有
iPadでの共有には sharePositionOrigin
パラメータを指定する必要があります。これを指定しないと、アプリがクラッシュする可能性があります。
Builder(
builder: (BuildContext context) {
return ElevatedButton(
onPressed: () => _onShare(context),
child: const Text('Share'),
);
},
),
// _onShareメソッド:
final box = context.findRenderObject() as RenderBox?;
await Share.share(
'check out my website https://example.com',
subject: 'Look what I made!',
sharePositionOrigin: box!.localToGlobal(Offset.zero) & box.size,
);